How to Create a Cryptocurrency: Coins vs. Tokens, Tech Matters, & Examples
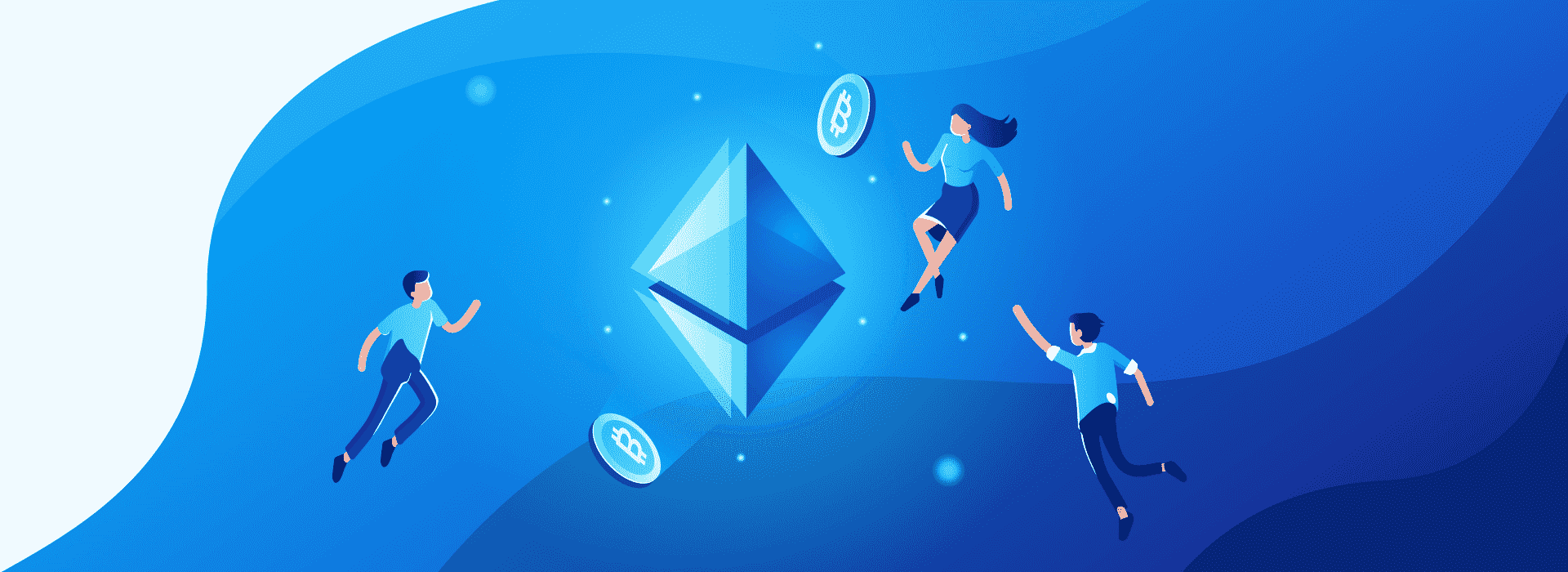
With the advancement of blockchain technology, more and more people have begun to wonder how to create a cryptocurrency.
In fact, there are a few major ways to do this.
This updated article will guide you along through the main technical and business aspects of new cryptocurrency creation. You will learn how coins and tokens differ, and which solutions can be used to make your own cryptocurrency, as well as:
- Define what cryptocurrency means in simple words
- Elaborate on how cryptocurrency works
- Pros & cons of cryptocurrency
- Ways to build your own cryptocurrency
- Step-by-step process to make a new cryptocurrency
- Price to pay to create your own crypto coin
Let’s get started with the details of how you can make your own cryptocurrency.
What is a Cryptocurrency?
Cryptocurrency is a new phenomenon in our world, and though it is more than 13 years old, it is still being misrepresented by many.
While some believe it to be a new form of money, others consider it just a buzzword. However, there is so much more to the story. Before diving into more complex concepts and defining how to make your own cryptocurrency, you should first know the answer to the question, “what is a cryptocurrency?”
Cryptocurrency is a decentralized digital currency that uses encryption techniques to regulate the generation of currency units and to verify the transfer of funds.
Anonymity, decentralization, and security are among its main features. Cryptocurrency is not regulated or tracked by any centralized authority, government, or bank.
Blockchain, a decentralized peer-to-peer (P2P) network, which is comprised of data blocks, is an integral part of cryptocurrency. These blocks chronologically store information about transactions and adhere to a protocol for inter-node communication and validating new blocks. The data recorded in blocks cannot be altered without the alteration of all subsequent blocks.
So, when did cryptocurrency come into being?
Even though virtual money became available long ago, Bitcoin is the first known and successful cryptocurrency holding the foremost position in the cryptocurrency market. According to Statista, as of today, there are over 10,000 types of cryptocurrencies, including the most popular ones like Bitcoin (BTC), Ethereum (ETH), and Tether (USDT) and the number is still growing.
All of the above information makes companies see the benefits of blockchain and makes them think about how to create a cryptocurrency.
How Does a Cryptocurrency Work?
As mentioned, cryptocurrency is an integral part of the blockchain. Distributed ledger technology is built on the consensus algorithms regulating the creation of new blocks. All participants in the P2P network have to accept a block for it to be registered in the blockchain. There are several types of consensuses with PoW (proof-of-work), PoS (proof-of-stake), DPoS (delegated proof-of-stake), and PoA (proof-of-authority) among the most popular.
Cryptocurrency is issued every time a new block is created and is used as a reward and incentive for blockchain participants taking part in the consensus mechanism and closing blocks, i.e. allocating their processing power, stakes of coins, and other resources to support the transparency and trust of blockchain and to verify new blocks. With this purpose, Bitcoin was created.
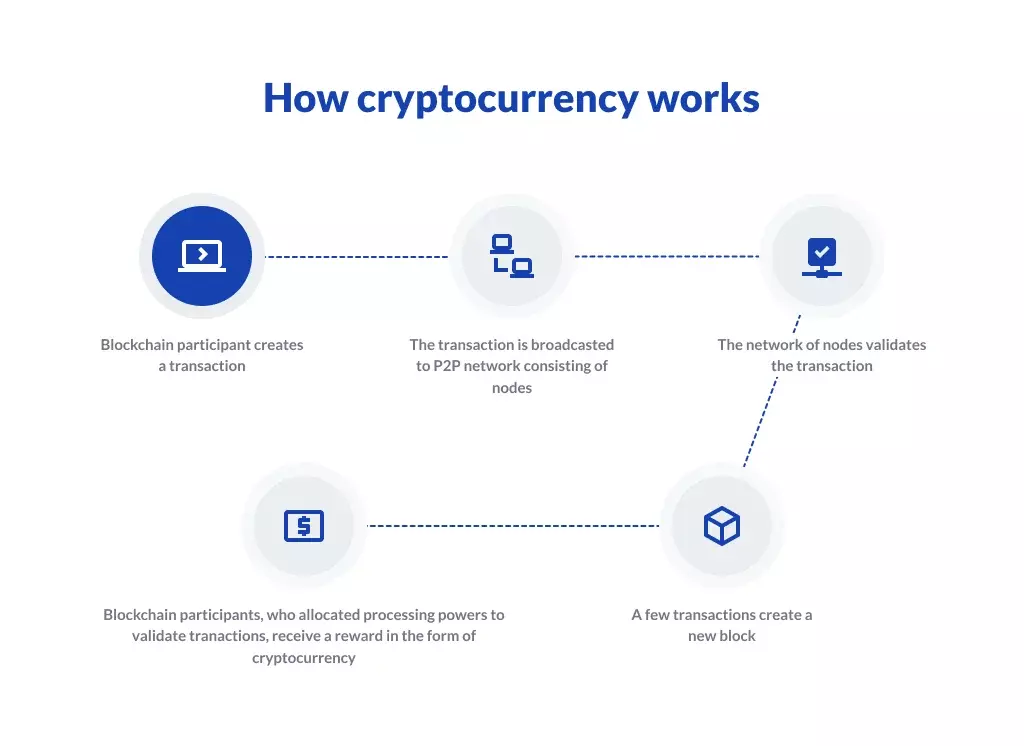
Cryptoholders can transfer cryptocurrency assets between wallets and blockchain addresses, exchange it for fiat money, or participate in cryptocurrency trading.
Everyone on the network can view transactions, while the identities of the people behind these public addresses remain anonymous, as they are encrypted by unique keys that connect an individual to an account.
Difference Between Coins and Tokens
Cryptocurrencies can be divided into two large subcategories – coins and tokens. While they are both cryptocurrencies, there is a difference between a coin and a token. Understanding their main concepts will help you figure out how to make your own cryptocurrency for specific business needs.
A coin operates on its own blockchain where all transactions occur.
Examples include Bitcoin, Ethereum, Neo, and Emercoin, all of which operate on a different blockchain. If you want to make your own coins, you need to first create your own blockchain.
A token works on top of an existing blockchain infrastructure, like NEO or Ethereum, which is used to verify transactions and make them secure. Tokens are often used like smart contracts, representing everything from physical objects to digital services.
Anyone can use Ethereum or Neo as the underlying technology to start a new cryptocurrency. The primary use for tokens is a security token offering (STO), which helps projects and startups fund operations through a crowdsale. This is the main reason why companies start considering the question of how to create a cryptocurrency in the first place.
Want to create a new cryptocurrency or implement a project with the help of blockchain technology?
Reach out to our team for a piece of free advice. We will elaborate on your project concept, explain to you all ins and outs of project implementation as well as estimate its cost and timeline.
Advantages and Disadvantages of Cryptocurrencies
If you are thinking about how to create a cryptocurrency, you first need to know the pros and cons. Read on to learn more about why cryptocurrencies are popular and why you should use cryptocurrency in your business operations.
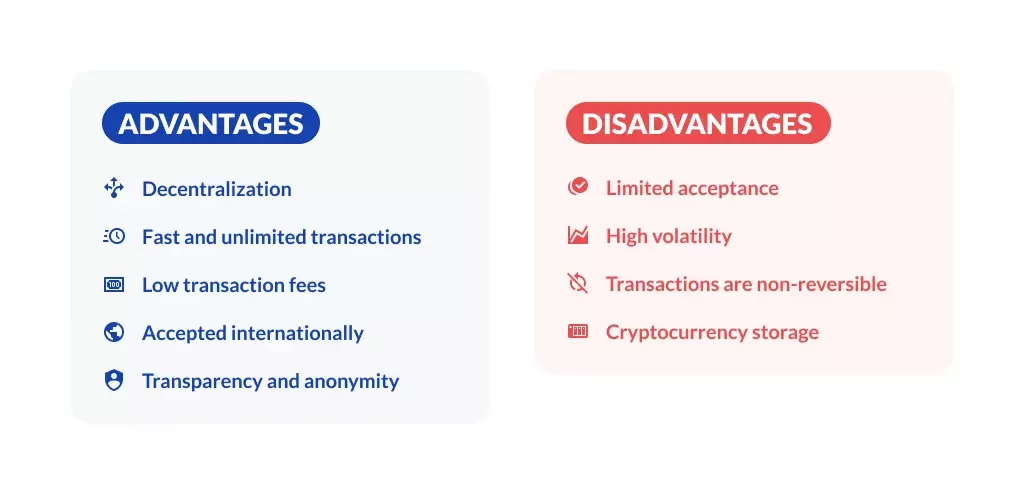
Advantages of cryptocurrencies are as follows:
- Decentralization
The main argument in favor of cryptocurrencies is their underlying technology - blockchain. This makes cryptocurrency independent from any authority and makes it so that no one can dictate the rules for cryptocurrency developers and owners.
- Fast and unlimited transactions
Fiat money transactions take a significant amount of time to be processed and settled. Your business will end up waiting days to receive money. With cryptocurrency, you can create an unlimited number of transactions and send it almost immediately to anyone with a crypto wallet, anywhere in the world.
- Low transaction fees
Banks and other financial institutions levy considerable transaction fees. This doesn’t mean that you don’t need to pay a fee for cryptocurrency transactions; however, the amount you need to pay is relatively small.
- Accepted internationally
The sender and the recipient of funds can be in different parts of the world and still exchange cryptocurrency. You can save money on currency conversion and the fees that always accompany international funds transactions.
- Transparency and anonymity
Thanks to the distributed nature of blockchains, every transaction is recorded and the records are immune to changes. At the same time, if a crypto address is not publicly confirmed, no one will know who made a transaction and who received the cryptocurrency.
All of the above should make companies think more seriously about how to make a cryptocurrency. However, there are some drawbacks you should also consider.
The disadvantages of cryptocurrencies are:
- Limited acceptance
Countries are very hesitant about granting any cryptocurrency their support. In everyday life, there are still limited possibilities for those who want to make purchases with cryptocurrency. So, rather than asking about how to create a cryptocurrency, people more often wonder how to use cryptocurrency at all.
- High volatility
Very often, users thinking about how to get started with cryptocurrency forget about an important factor - high volatility. The cryptocurrency market is not stable, with frequent ups and downs even for famous cryptocurrencies like Bitcoin. It is highly risky to invest in cryptocurrency, as you never know whether it will be a profitable investment or not.
- Transactions are non-reversible
Mistakenly entering an incorrect cryptocurrency address may cost you money. There is no way to reverse a transaction. You may send a request for a refund, but if it is declined, be ready to say goodbye to your money.
- Cryptocurrency storage
You’ve probably read horrible stories about cryptocurrency owners who lost their devices, forgot the private key, and could not access their cryptocurrency fortunes. These sorts of situations can happen to anyone, so anyone can lose their money accidentally.
These benefits and drawbacks should be taken into account when considering how to create a cryptocurrency that will facilitate your business goals. You need to decide what the purpose of cryptocurrency creation is for your company.
How to Create a Cryptocurrency: Technical Matters
So, you want to know how to create a cryptocurrency?
You can start a new cryptocurrency by creating an entirely new blockchain with a coin or by forking an existing one and creating a token.
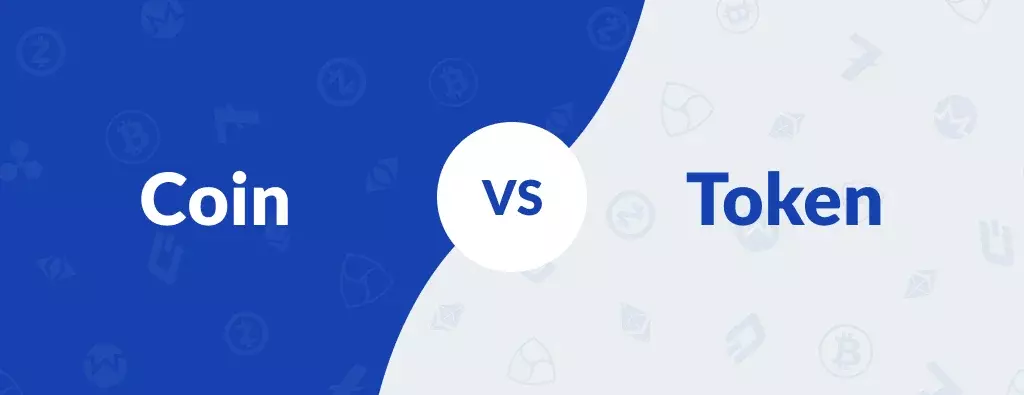
You can find many tutorials online about how to become a cryptocurrency creator, though all of them require at least basic coding skills and an in-depth understanding of blockchain.
Two Ways to Create a Cryptocurrency
1. Creating a coin
This option is not suitable if you are looking for an easy and fast way to create your own cryptocurrency free of charge. You need to be an experienced professional in decentralized technologies or have someone who is willing to take on the role of the technology expert.
The process of coin creation may take as little as 5 minutes. You can just copy the code of Bitcoin, add a new variable, or even change the value of something, and that’s it - you have your blockchain and coin. However, you need to understand the code and how to change it, which requires extensive coding skills.
Another issue is to maintain, support, and promote the coin, as you have to create the whole logic of blockchain to launch your coin. Hiring a team of professionals to handle the task would save more time, but you would have to pay custom software development services. If you can afford to allocate a budget toward creating and supporting your own blockchain, go for it.
2. Creating a token
This is a more feasible way to become a currency creator. While having complete control over the blockchain may sound like a great idea, this has certain drawbacks like increased development time, significant spending, and much more.
Fork cryptocurrency is created on top of an existing blockchain by utilizing the trust, popularity, and consensus mechanism of the underlying technology. When you build a token on top of a strong blockchain, like Ethereum, your atoken runs on a secure network that is protected from fraudulent attacks. Token creation is less costly in terms of money and time, as you utilize the existing decentralized blockchain architecture and implemented consensus mechanisms.
In the table below, you will see the pros and cons of building coins or tokens:
Coin | Token |
---|---|
Requires the creation of a new blockchain | Can be built on the existing and trusted blockchains |
In-depth knowledge of blockchain and coding skills are required | Relatively easy to create with open source code |
Blockchain development require more investment | Token creation is easier, faster, and more cost efficient |
Popular Solutions for Making a Cryptocurrency
There are a few blockchains that provide the means for creating a token. Ethereum, NEO, and EOS are the most popular tools and are relatively easy to use. When talking about how to create a cryptocurrency, you will probably hear about one or all of these solutions.
1. Ethereum
Ethereum became the first blockchain to offer token creation service. It provides an exceptional level of trust due to its maturity and strong position on the cryptocurrency market. All tokens built on Ethereum use the ERC-20 standard. The documentation is well written and organized, making the development process easier. A token on Ethereum can only be written in Solidity (its own programming language), but with the HTTP API you can create dApps in any language.
2. NEO
NEO blockchain is aimed at the smart economy and utilizes the NEP-5 standard. Unlike Ethereum, you can use almost any high-level programming language, including C#, Java, Python, and Kotlin to create your own token on top of it. HTTP API is available for interaction with the blockchain.
3. EOS
EOS tokens use the EOSIO.Token standard and can be created with C++ or any other language that compiles into WebAssembly. The blockchain offers great scalability, a vast number of transactions per second, and cost efficiency due to the lack of a truncation fee. The name of this blockchain often arises when discussing how to create a cryptocurrency.
The table below presents the main aspects of creating a new cryptocurrency with Ethereum, NEO, and EOS.
Ethereum | NEO | EOS | |
---|---|---|---|
Programming language | Solidity (Ethereum’s own programming language) | Almost any high-level programming language, including C#, Java, VB.Net, F#, Kotlin, Python, etc. | C++ and any language that compiles into WebAssembly (WASM) |
Token standard | ERC-20 | NEP-5 | EOSIO.Token |
Virtual Machine | Native Virtual Machine | Native Virtual Machine | Native Virtual Machine |
Wallets | A lot of options | A lot of options | A lot of options |
Hardware wallet | Yes | No | Yes |
Consensus | PoW (proof-of-work) before the switch to PoS (proof-of-stake) | dBFT (Delegated Byzantine Fault Tolerance) | DPoS (Delegated proof-of-stake) |
Primary sphere of use | Smart contracts | Smart economy, digital identity, digital assets | Smart contracts |
Number of transactions per second | 15 transactions/second |
10,000 transactions/second |
3,000+ transactions/second |
The vast majority of blockchains that can be used as an underlying network for a new token have broad communities and detailed documentation. However, you need to be an expert in programming to understand it all and use the knowledge for further development.
While it is possible to create a new cryptocurrency all by yourself following any "make your own cryptocurrency" tutorial, only a team of professionals can choose the best stack of technologies and finish the development process in record time.
How to Make a Cryptocurrency: Key Business Processes
Before diving headfirst into cryptocurrency development, you need to think the whole process through. We will explain how to make a cryptocurrency by following seven main steps:
1. Define your own coin idea
Creating a cryptocurrency may be fun, but in real-life business you have to develop a strategic plan. Define not only how to create a cryptocurrency, but what problem you want to solve with your dApp and what audience it will target.
Professional business analysis services can help with this critical step. Maybe you want to remove a bank or other middleman during transactions, or create a life-changing healthcare solution.
To make customers interested in your token during ICO/STO, create a valuable proposition. You can find a lot of guides on how to create an ICO, but without value, even the greatest idea remains just an idea.
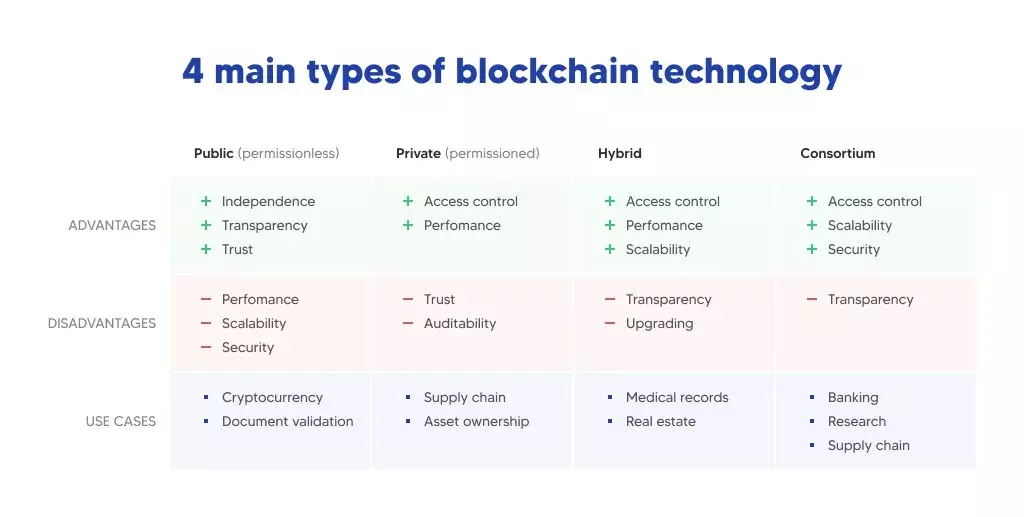
If you are interested in developing a crypto coin, you have a few options to choose from. The most complex option is to create your own coin and blockchain architecture from scratch. Alternatively, it is possible to base your cryptocurrency on an existing blockchain platform simply by adjusting its code. Moreover, you can set up a new crypto coin on the existing blockchain. In most cases, you need to hire a blockchain developer for faster and more satisfying results.
The following are be use cases and purposes for creating cryptocurrency:
- Transferring money in a secure and encrypted manner
- Crypto coins for storing your wealth
- Supporting smart contract
- Verification of information and a massive amount of data
For instance, Dogecoin crypto coin, was developed at the peak of meme popularity. In contrast, IMPT crypto coin is a new token that seeks to create an ecosystem for brands that want to reduce their carbon footprint.
Please note: Building your own coin is a rather simple task. Supporting and growing it over time, however, is far more challenging and vital.
2. Select a blockchain platform
The initial step of actual crypto coin creation lies in selecting the blockchain platform on which it will be based. This allows the system to track the records and make cryptocurrency transactions accountable. A blockchain platform works according to a consensus mechanism - a communication protocol for evaluating and proceeding transactions.
You can use Ethereum, Binance Smart Chain, EOS, and NEM as the most popular and widely used existing blockchain platforms.
3. Choose the right development team
While you can try to decide how to create a cryptocurrency in the best way, confiding in an experienced software development company is more efficient for your business.
As we’ve already mentioned, it is vital that you choose the right team by applying the right software vendor selection criteria to bring your idea to life. Consider hiring experienced professionals who know their way around the blockchain and cryptocurrency world. Though the services won’t be cheap, you will be able to avoid the need for more work in the future.
4. Create rules for smart contracts
A smart contract will have a significant impact on your project. Smart contracts are like traditional contracts, except for the fact that they are digital, operate on the blockchain, have pre-established rules, cannot be changed, and are executed automatically. You can create your own rules representing the main idea behind ICO/STO.
Cryptocurrency Сreation: Key Business Processes
5. Hire an external audit company
Making a cryptocurrency legal depends on the location and its jurisdiction. Therefore, ensure that you create your crypto coins in a crypto-friendly country. To do this, it is vital to involve a legal advisor to follow the rules and capture all aspects correctly in the white paper of crypto coins.
Trust in ICO/STO has lessened as many initial coin offerings have turned out to be fraudulent scams. Investors are picky about the projects they choose to invest their funds in. Hiring an external audit company will make your cryptocurrency legal. ICO/STO security audits must be carried out by a trusted company with an established reputation of credibility.
6. Dedicate time to crafting a white paper
Investors judge projects by, and get their first impressions from, provided white papers. If this does not reveal the value behind your idea, they may turn their backs on you. A well-written white paper helps you cross the invisible bridge from failure to a successful ICO/STO.
The white paper should answer the following questions:
- What is the problem and why is a new solution needed?
- What is your company planning to spend ICO/STO funds for?
- When will tokens will be released, how many, and on which exchanges?
- What roadmap will the project follow?
- Who is on your team, what experience do they have, and how can they bring value to the project?
7. ICO promotion
After accomplishing all the steps mentioned above, it is time to move on to ICO/STO marketing. Social media, press media, guest blogging, email marketing, and overall promotion are all great places to start. You can use all the traditional marketing tools, but choose them wisely as you need to clearly define what gives cryptocurrency value.
8. Create a strong community and support it
Do you know what Bitcoin, Ethereum, Ripple, and NEO have in common?
All of these blockchains have active and strong communities. Choose the right channels to communicate with the community members, try to answer all their questions, and provide timely updates regarding project development. You need a dedicated team to manage your community 24/7.
The information provided above describes how to create a cryptocurrency that will earn a high place on the market. Follow the steps to make sure you not only know how to make your own cryptocurrency, but also to ensure it will be trusted and supported on the cryptocurrency market.
How to Make Your Own Cryptocurrency: Example
The decision-taking of how to create a cryptocurrency wouldn’t be complete without an example. The programming behind making a unique token can be very complex. However, down below we have the structure of any basic cryptocurrency or token. This will help you create your own Ethereum token. A more complete cryptocurrency source code can be found here
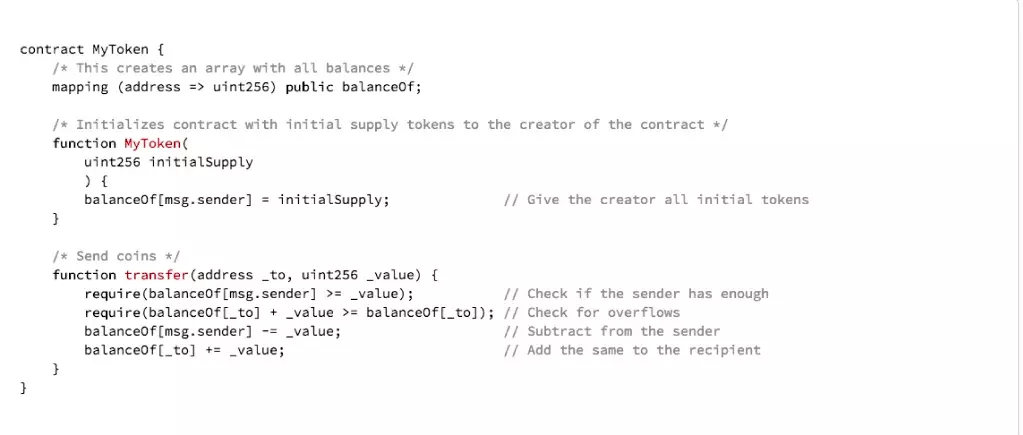
Create an Ethereum Token
In order to create your own cryptocurrency, you will need to use the ETH wallet app that can be found and downloaded here. When you open the wallet app, at the top right corner, you will see a button as depicted below, “Deploy New Contract”, click it.
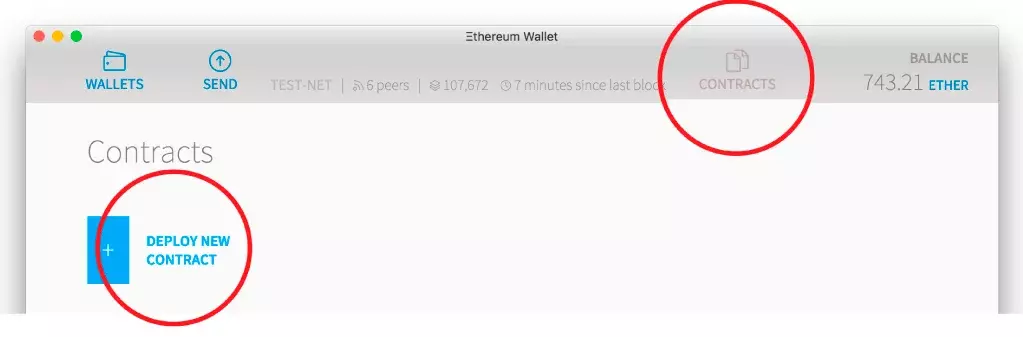
Once you click, a Solidity Contract Source Code field will pop up. Enter this code in the field that pops up.
contract MyToken { | |
/* This creates an array with all balances */ | |
mapping (address => uint256) public balanceOf; | |
} |
The phrase “mapping” stands for an associative array, which associates balances with addresses. All addresses are in hexadecimal format. “Public”, which is bolded, means that anyone will be able to see balance. After you add the line of code to the Solidity field, your screen should look like this.
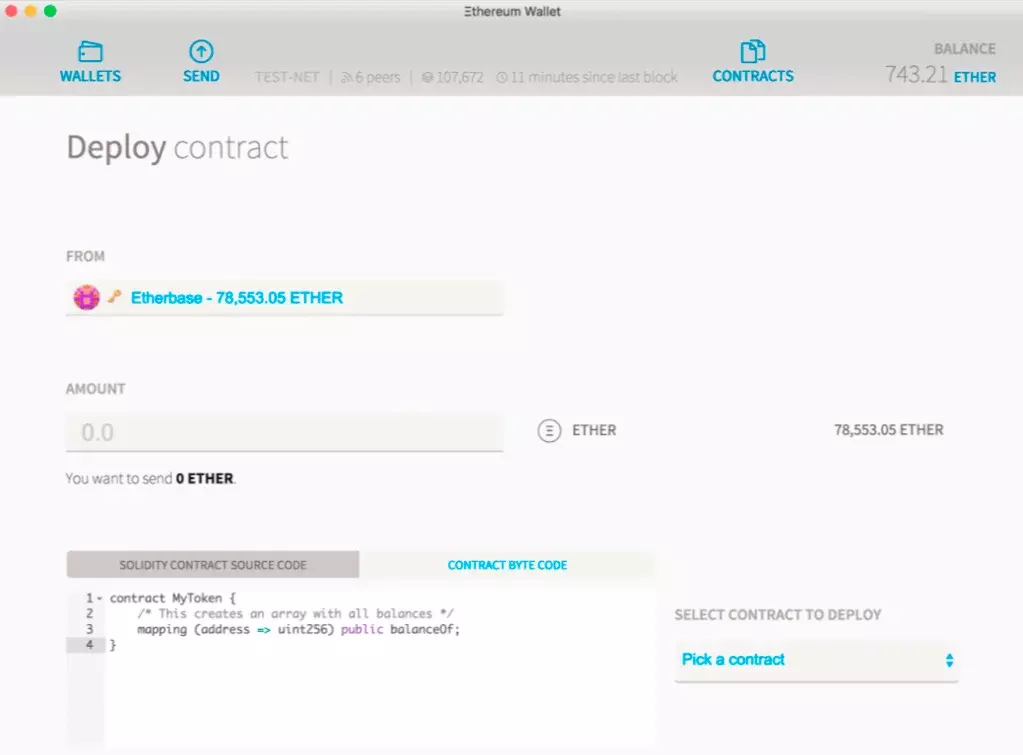
However, this doesn’t mean that your cryptocurrency has actually been created. What you need to do now is add another line of code under line 4 in the Solidity code box as follows:
function MyToken() { | |
balanceOf[msg.sender] = 21000000; | |
} |
Your initial token supply will be 21 million. However, you can easily set this amount to anything you like. Let’s take a glance at the right side of the application. Click “Select Contract to Deploy” and a drop-down window will open. Click “MyToken.”
How to Move Your Cryptocurrency
After following the aforementioned steps, you will have a smart contract that is linked to a token. However, you can’t move them yet. Let’s fix that by adding the following code below the last bracket in the Solidity field.
/* Send coins */ | |
function transfer(address _to, uint256 _value) { | |
/* Add and subtract new balances */ | |
balanceOf[msg.sender] -= _value; | |
balanceOf[_to] += _value; | |
} |
What we have here is relatively self-explanatory. We can send tokens and values will be subtracted or added where necessary. However, how do we handle people that want to send more than they possess? In order to stop a contract from executing itself under such conditions, we add another line of code to the Solidity box.
function transfer(address _to, uint256 _value) { | |
/* Check if sender has balance and for overflows */ | |
require(balanceOf[msg.sender] >= _value && balanceOf[_to] + _value >= balanceOf[_to]); | |
/* Add and subtract new balances */ | |
balanceOf[msg.sender] -= _value; | |
balanceOf[_to] += _value; | |
} |
We must now add some basic information into the Solidity field pertaining to our contract. Proceed like so:
/* Initializes contract with initial supply tokens to the creator of the contract */ | |
function MyToken(uint256 initialSupply, string tokenName, string tokenSymbol, uint8 decimalUnits) { | |
balanceOf[msg.sender] = initialSupply; // Give the creator all initial tokens | |
name = tokenName; // Set the name for display purposes | |
symbol = tokenSymbol; // Set the symbol for display purposes | |
decimals = decimalUnits; // Amount of decimals for display purposes | |
} |
Creating Events and Deploying Your Cryptocurrency
After we have gone through all of the steps mentioned above, we now need to create something known as “Events.” These are essentially empty functions that allow Ethereum wallets to monitor the activities of a contract. Remember that every event must start with a capital letter. In order to declare an event, the following code must be added:
event Transfer(address indexed from, address indexed to, uint256 value);
As well, within the “Transfer” function, add this:
/* Notify anyone listening that this transfer took place */
Transfer(msg.sender, _to, _value);
After you do this, your token is essentially good to go, all you have to do is deploy it.
Navigate over to the “contracts tab” and hit “deploy new contract.” Following this, copy and paste the token source found here. If there is an issue, Solidity will notify you. On the right side of the application you will see a few parameters such as _supply, _name, _symbol, _decimals, you can tweak and alter all of these to your liking.
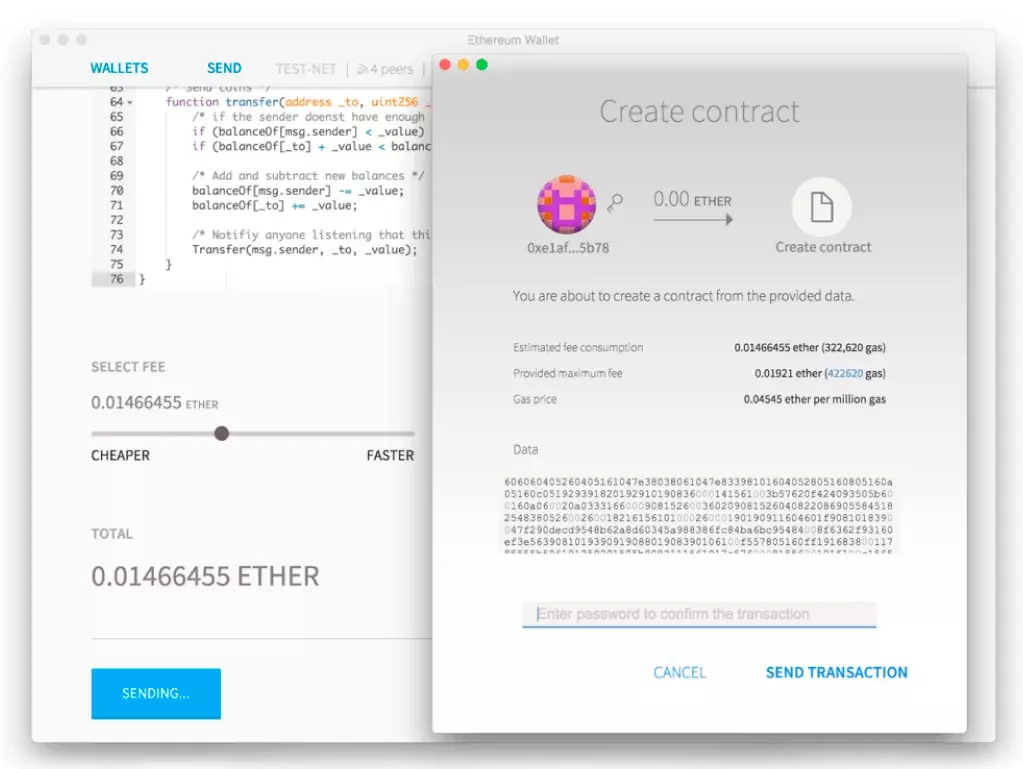
After tweaking all of this, you can then set a fee for your token. You’re good to go and you can deploy your cryptocurrency. Check out the image to the right for more information. The password field will prompt you to enter the password that you set when you downloaded the wallet app.
Now you know how to make a cryptocurrency in practice, and you can even send it to your friends! All you have to do to make that happen is to navigate to the send tab, and send tokens to anybody you know. Of course, your tokens will not have any value because they aren’t yet tied to any product or service, which means that nobody will buy them and they won’t be listed on Coinmarketcap. However, understanding blockchain technology isn’t that hard, is it?
If you want to create a full-fledged cryptocurrency, it’s better to turn to the professionals.
Five Best Cryptocurrencies on the Market
How many cryptocurrencies exist?
According to Coinmarketcap, there are 18,000 cryptocurrencies on the market and the number is still growing.
While it is impossible to name the single best cryptocurrency on the market, below we have listed the most popular ones. They are all worth your attention.
1. Bitcoin
This was the first cryptocurrency to be created. To this day, Bitcoin is still at the top of the game. Bitcoin has a 40% share of the total cryptocurrency market, and there is no indication that it will stop growing in the years to come. As of today, the market capitalization of Bitcoin accounts for $834 billion.
2. Ether
Ether matches Bitcoin in popularity and recognition. Launched in 2015, Ether has shown steady growth over the last few years with a market share of 11.022%. The underlying blockchain, Ethereum, is used by developers to create new tokens. The market capitalization of Ether constitutes $372 billion and continues to grow.
3. Tether (USDT)
Tether is a stable coin meaning it is stabilized by fiat money including the US dollar and the Euro. In theory, it holds a value that would equal one of the currencies mentioned. As a result, Tether’s value is more consistent compared to other cryptocurrencies and investors who want to avoid high coin volatility favor the Tether coin. These days, Tether’s market capitalization has reached over $80 billion.
4. Litecoin (LTC)
Litecoin (LTC) has been operating since 2011 and is among the best cryptocurrencies to follow, aside from Bitcoin. This cryptocurrency is based on an open-source payment network without central control and utilizes script as a PoW (proof-of-work). Despite the fact that Litecoin resembles Bitcoin in some ways, it has a quicker block generation rate. Thus, Litecoin confirms transactions faster, and more and more merchants are accepting the cryptocurrency. These days, Litecoin has a market capitalizationof over $8 billion.
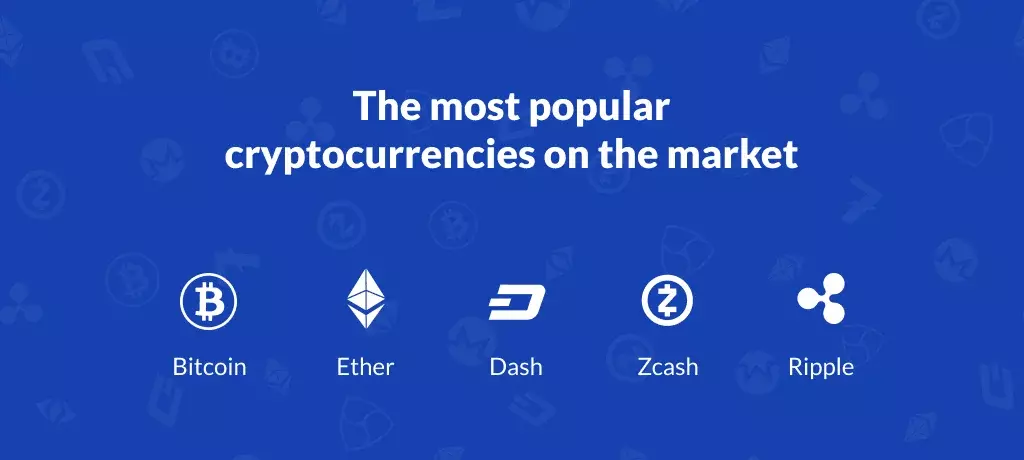
5. Binance Coin (BNB)
This cryptocurrency was launched in 2017 for assisting trading on Binance, one of the key crypto exchanges globally. This version of cryptocurrency allows its users to trade and pay fees on Binance with a significant discount. These days, it can also be used for trading, payment, booking travel events, as well as exchanging other cryptocurrencies like Bitcoin or Ethereum. The market cap of Binance Coin is over $68 billion.
The information provided above indicates that blockchain and distinct types of creation on its basis remain one of the top technologies on the market.
Companies see value in the advantages that decentralized technologies offer: security, transparency, anonymity, and fast transactions. Businesses can’t stop thinking about how to create a cryptocurrency and utilize it in everyday business operations.
Want to know more about blockchain and what the future entails for this technology?
How to Create a Cryptocurrency: Costs
You can create a cryptocurrency to raise money for your project (ICO), to use in your applications, or both.
In either case, the process is comprised of various steps you should take. If you are not ready to take on the challenge, you need a qualified team to accompany you along this bumpy road.
If you are thinking about how to make your own cryptocurrency, we are sure that you would like to know how much it might cost as well. Let's find out.
The price of cryptocurrency creation depends on the specific needs of a project and whether you choose to create a new cryptocurrency from scratch or use an existing blockchain as the underlying technology. It is up to you to decide how to create a cryptocurrency.
Usually, the cost of cryptocurrency development includes the following expenses, but may vary greatly:
Stage | Time | Costs |
---|---|---|
Development | Varies from project to project (from 15 minutes to 5-6 months) |
$1,000-$100,000+ |
External Audit (ICO Security Audit) | 30 days | $3,000-$10,000 |
White Paper and Documentation | 24-50 hours (1-2 weeks of full-time work) |
$5,000 to $7,000 (~$500 per page) |
Promotion | 1 week - 30 days | $10,000 per week |
Coin Placement | 1-2 weeks | $5,000+ |
Is it Worth it to Create Your Own Cryptocurrency?
Technical issues are the hardest part of creating your own cryptocurrency. You can easily find tutorials on how to create your own cryptocurrency in 15 minutes or how to make a cryptocurrency without coding, but in reality, creating cryptocurrency is not easy. You need to have extensive experience in blockchain programming to accomplish the goal. Only qualified specialists have the knowledge and experience to walk you through this challenging task.
You need to have a solid purpose and goal in new cryptocurrency creation to stand out since there are over 18,000 cryptocurrencies while only 90 are popular and used widely. Still, there is also a way to improve some systems and resolve new issues that can be encountered with the help of blockchain technology and/or new cryptocurrency.
As well, you need to have extensive experience in blockchain programming to accomplish your goal. If not, you should hire qualified specialists who have the knowledge and experience to walk you through this challenging task.
Need to make your own cryptocurrency?
Contact us and we will happily help you to decide how to create a cryptocurrency and assist you in this task.